Batch File Random Letter Generator
Perhaps this is what you want? TEST.BAT @echo off set charPool=abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ set charLen=62 (for /L%%a in (1,1,%1) do ( set permutation= call:makePermutation%%a )) textfile.txt goto:EOF:makePermutation level setlocal EnableDelayedExpansion set lastPermutation=%permutation% for /L%%i in (1,1,%charLen%) do ( set permutation=!lastPermutation!!charPool:%%i,1! If%1 gtr 1 ( set /A newLevel=%1-1 call:makePermutation!newLevel! ) else ( echo(!permutation!
Give More Feedback
) ) exit /B The batch file must be started with a number as parameter which is the unit length. For example on using TEST.BAT 1 the text file textfile.txt contains 62 lines. Note that TEST.BAT 2 generates 3906 combinations (strictly speaking, permutations in statistical sense) from 0 to ZZ, and TEST.BAT 3 generates 242234 combinations from 0 to ZZZ! Calculation example for estimating the number of strings in text file (size of file): Running TEST.BAT with 5 as parameter produces 62 ^ 5 + 62 ^ 4 + 62 ^ 3 + 62 ^ 2 + 62 ^ 1 = 931.151.402 strings in text file. Well I tryed to plug in what you just gave me into a batch file. Gave it a unit of 2.
I'm having a hard time figuring out how to make a password generator with random letters. You make a letter password generator in batch? The batch file.
When I ran it, all it did was make the text file then stop and did not print the combinations not sure where I could add a pause in to see whats going on but the code it self looks right as to what I want. What i would like is to be able to set a unit for 8 but it starts at 1 and then works up to it. I do know that it would end up with well over 200 trillion combinations if the unit was set to 8. What would be amazing is if there was a way to right this to the ram on my computer and then at the end of – Jun 14 '12 at 17:48. Here is an iterative solution that is much faster. No need for CALL. Each permutation is only generated once.

I was able to generate up to length 4 with over 15 million permutations in less than 5 minutes. @echo off setlocal enableDelayedExpansion set chars=abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ set maxPos=61 del output.txt 2nul prior.txt echo(' for /l%%I in (1 1%1) do ( new.txt ( for /f%%A in (prior.txt) do for /l%%N in (0 1%maxPos%) do echo(%%A!chars:%%N,1! ) type new.txtoutput.txt move /y new.txt prior.txt nul ) del prior.txt.
I am wanting to create a password generator which uses a list of pre-generated passwords using batch. The following script I am trying to adapt. I want the system to automatically generate a password using the prefix LONDON Also I want a section where we can can change the word if possible. Can anyone assist? Also, it needs to generate a password from LONDON01 to LONDON100. @echo off:Start2 cls goto Start:Start title Welcome to my Password Generator echo I will make you a new password. Echo Please write the password down somewhere in case you forget it.
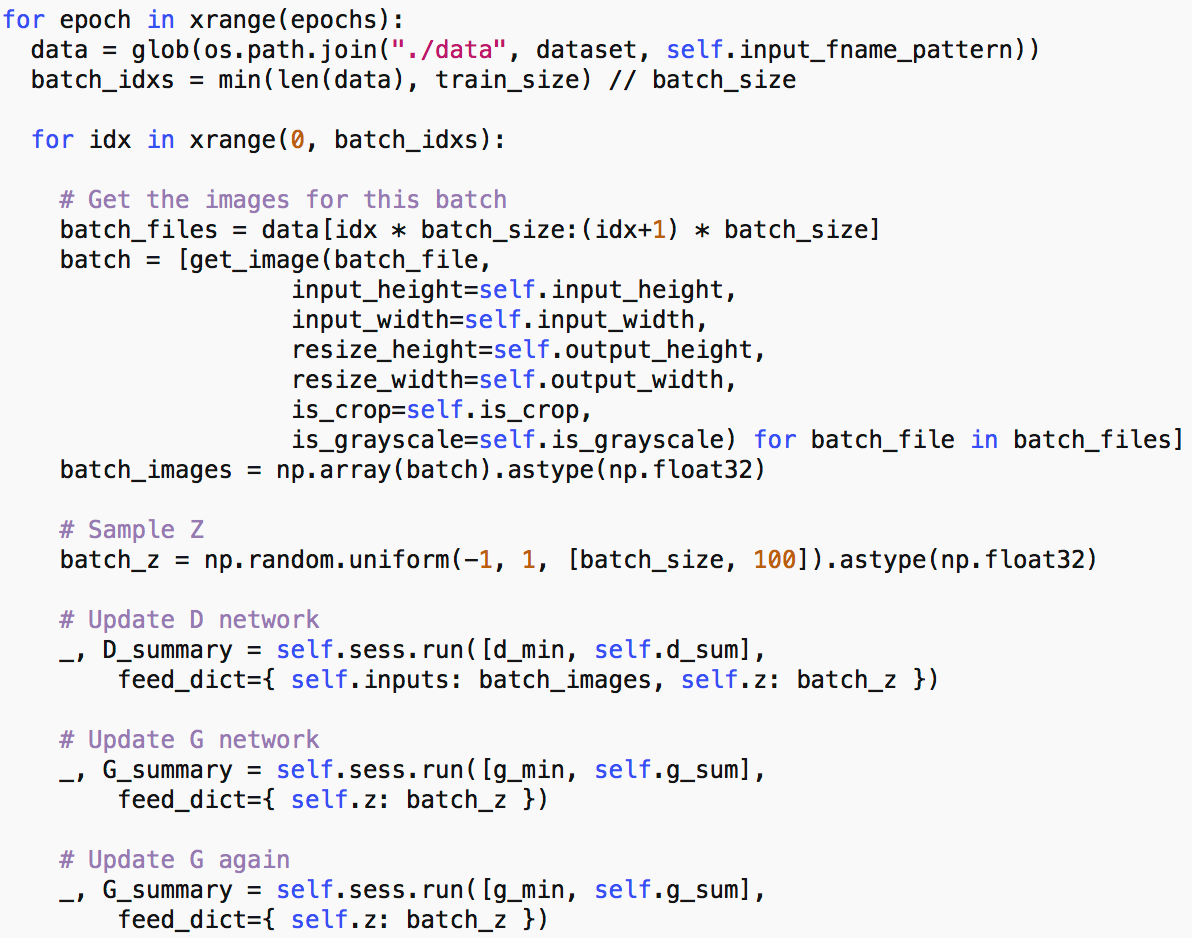

Echo -- echo 1) 1 Random Password echo 2) 5 Random Passwords echo 3) 10 Random Passwords echo Input your choice set input= set /p input= Choice: if%input%1 goto A if NOT goto Start2 if%input%2 goto B if NOT goto Start2 if%input%3 goto C if NOT goto Start2:A cls echo Your password is%random% echo Now choose what you want to do. Echo 1) Go back to the beginning echo 2) Exit set input= set /p input= Choice: if%input%1 goto Start2 if NOT goto Start 2 if%input%2 goto Exit if NOT goto Start 2:Exit exit:B cls echo Your 5 passwords are%random%,%random%,%random%,%random%,%random%. Echo Now choose what you want to do. Echo 1) Go back to the beginning echo 2) Exit set input= set /p input= Choice: if%input%1 goto Start2 if NOT goto Start 2 if%input%2 goto Exit if NOT goto Start 2:C cls echo Your 10 Passwords are%random%,%random%,%random%,%random%,%random%,%random%,%random%,%random%,%random%,%random% echo Now choose what you want to do. Echo 1) Go back to the beginning echo 2) Exit set input= set /p input= Choice: if%input%1 goto Start2 if NOT goto Start 2 if%input%2 goto Exit if NOT goto Start 2. If you want a fairly decent 'random', then I would suggest a fast program in C or something else (which can be distributed to your office systems). But, if you are stuck on cmd then: @echo off set minimum=0 set maximum=100 set pre=LONDON:A set randnumber=%random% if%randnumber% GEQ%minimum% ( if%randnumber% LEQ%maximum% ( echo%pre%%randnumber% goto END ) ) goto A:END Output Examples: Shouldn't be too hard to adjust that to work for your situation.
Edit: As a supplement, I wanted to speak to this as many others have: This is an extremely insecure way to generate passwords and really shouldn't be used for anything outside of locking a phone, perhaps even not that. A true password generator should have 'salt' that is randomly generated for each password and is always unique. Brute force on your password set (LONDON0 - LONDON100) would take seconds on a mid-line computer. Instead, you could use Python, something simple like: import string, random def genPass(prefix='LONDON', size=8, chars=string.asciiuppercase + string.asciilowercase + string.digits + '@!&%$'): return prefix + '.join(random.choice(chars) for i in range(size)) print genPass Which gets you a much more random, or at least unique, password set. If you want to change anything, just call genPass(prefix='NOTLONDON', size=2) instead, for example.
This should fix your existing batch file so it works. @echo off set 'prefix=LONDON':Start2 cls goto Start:Start title Welcome to my Password Generator echo I will make you a new password. Echo Please write the password down somewhere in case you forget it. Echo -- echo 1) 1 Random Password echo 2) 5 Random Passwords echo 3) 10 Random Passwords echo Input your choice set input= set /p input= Choice: if '%input%.' Goto:Start if%input%1 goto A if%input%2 goto B if%input%3 goto C goto:Start:A cls echo Your password is%prefix%%random%:reprompt1 echo Now choose what you want to do. Echo 1) Go back to the beginning echo 2) Exit set input= set /p input= Choice: if '%input%.'
Goto:reprompt1 if%input%1 goto Start2 if%input%2 goto Exit goto:reprompt1:Exit goto:EOF rem exit:B cls echo Your 5 passwords are%prefix%%random%,%prefix%%random%,%prefix%%random%,%prefix%%random%,%prefix%%random%.:reprompt5 echo Now choose what you want to do. Echo 1) Go back to the beginning echo 2) Exit set input= set /p input= Choice: if '%input%.' Goto:reprompt5 if%input%1 goto Start2 if%input%2 goto Exit goto:reprompt5:C cls echo Your 10 Passwords are%prefix%%random%,%prefix%%random%,%prefix%%random%,%prefix%%random%,%prefix%%random%,%prefix%%random%,%prefix%%random%,%prefix%%random%,%prefix%%random%,%prefix%%random%:reprompt10 echo Now choose what you want to do. Echo 1) Go back to the beginning echo 2) Exit set input= set /p input= Choice: if '%input%.' Goto:reprompt10 if%input%1 goto Start2 if%input%2 goto Exit goto:reprompt10 If you need something different, just let me know. Edit: I just noticed the comment indicating you need the results to be in the range of LONDON01 to LONDON100, so I modified the batch file to do that: @echo off set 'prefix=LONDON':Start2 cls goto Start:Start title Welcome to my Password Generator echo I will make you a new password. Echo Please write the password down somewhere in case you forget it.
See More On Stackoverflow
Echo -- echo 1) 1 Random Password echo 2) 5 Random Passwords echo 3) 10 Random Passwords echo Input your choice set input= set /p input= Choice: if '%input%.' Goto:Start if%input%1 goto A if%input%2 goto B if%input%3 goto C goto:Start:A cls call:getpws 1 call:showpws 1:reprompt1 echo Now choose what you want to do. Echo 1) Go back to the beginning echo 2) Exit set input= set /p input= Choice: if '%input%.'
Goto:reprompt1 if%input%1 goto Start2 if%input%2 goto Exit goto:reprompt1:Exit set 'pw1=' set 'pw2=' set 'pw3=' set 'pw4=' set 'pw5=' set 'pw6=' set 'pw7=' set 'pw8=' set 'pw9=' set 'pw10=' set 'pwcount=' set 'pwindex=' set 'pwmessage=' set 'pwresult=' goto:EOF rem exit:B cls call:getpws 5 call:showpws 5:reprompt5 echo Now choose what you want to do. Echo 1) Go back to the beginning echo 2) Exit set input= set /p input= Choice: if '%input%.' Goto:reprompt5 if%input%1 goto Start2 if%input%2 goto Exit goto:reprompt5:C cls call:getpws 10 call:showpws 10:reprompt10 echo Now choose what you want to do. Echo 1) Go back to the beginning echo 2) Exit set input= set /p input= Choice: if '%input%.' Goto:reprompt10 if%input%1 goto Start2 if%input%2 goto Exit goto:reprompt10:getpws set pwcount=%1 rem this step (clear) not totally necessary. Call:clearallpws for /L%%f in (1,1,%pwcount%) do call:get1pw%%f goto:EOF:clearallpws for /L%%f in (1,1,10) do call:clearpw%%f goto:EOF:clearpw rem clear password numbered by%1 set pwindex=%1 for /F 'usebackq delims='%%g in (`echo set 'pw%pwindex%='`) do%%g goto:EOF:get1pw set pwindex=%1 rem get a random number, add a leading 0 to make sure it is at least 2 digits long set pwresult=0%random% rem keep the last two digits set 'pwresult=%pwresult:-2,2%' rem this will now be something from 00 to 99 rem now add 1 set /a pwresult+=1 rem this will now be a number from 1 to 100. Add leading '0' for numbers below 10 if%pwresult% LEQ 9 set 'pwresult=0%pwresult%' rem this will now be a number from 01 to 100.
Insert the prefix set 'pwresult=%prefix%%pwresult% rem now assign it to pw(n) variable. And return for /F 'usebackq delims='%%g in (`echo set 'pw%pwindex%=%pwresult%'`) do%%g goto:EOF:showpws set pwcount=%1 set 'pwmessage=Your%pwcount% passwords are:%pw1%' if%pwcount% EQU 1 set 'pwmessage=Your password is:%pw1%' for /L%%g in (2,1,%pwcount%) do call:append1pw%%g echo%pwmessage% goto:EOF:append1pw set pwindex=%1 for /F 'usebackq delims='%%h in (`echo set 'pwmessage=%pwmessage%,%%pw%pwindex%%%'`) do%%h goto:EOF.