Arduino Software Interrupt
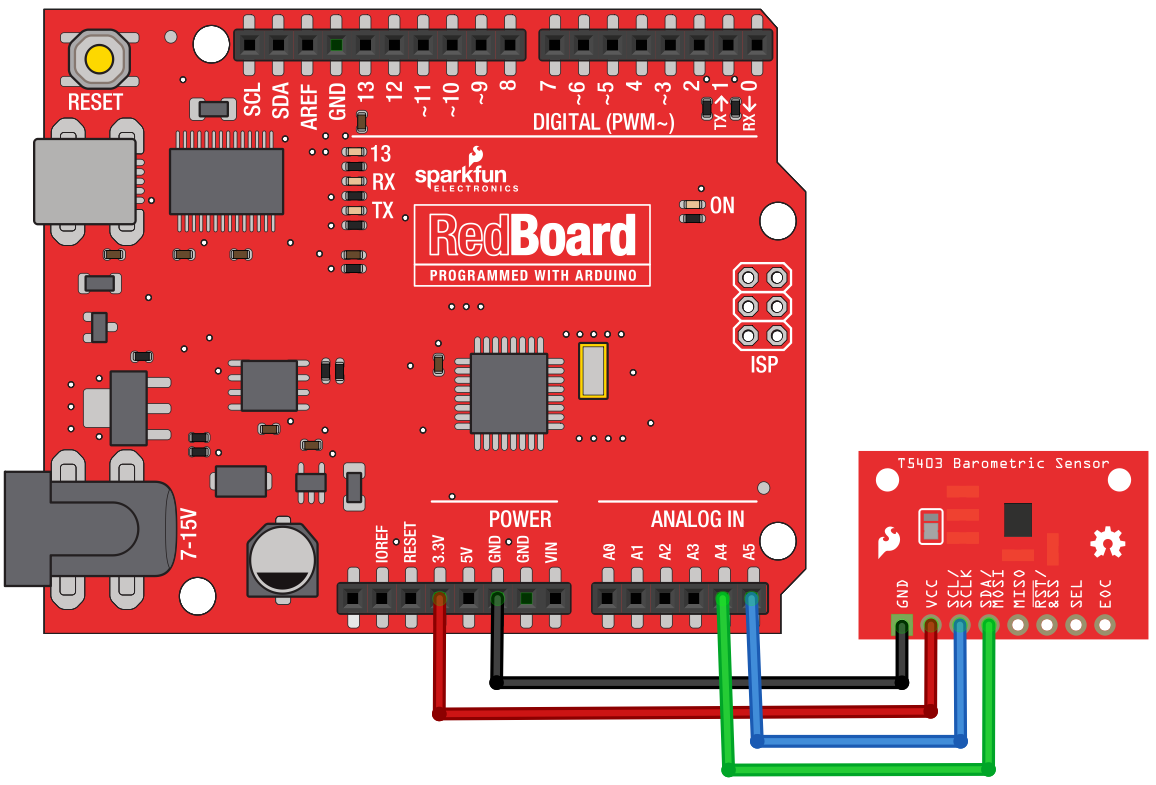
Well, as it turns out, your modifications are actually ones with pretty serious ramifications for interrupt service routines! Serial.println in the Arduino codebase is very similar to printf, which has some known re-entrance problems. It’s generally not a good idea to call printf style functions from interrupt service routines as a result - that’s why I used an LED in this example to show when the ISR fires.
Hi everybody! So recently i was working on a Project where i wanted to meassure the RPM of one of the Wheels on my Car, and from that calculate the Speed, keep.
If you absolutely have to have serial output in your program in response to an ISR, I would recommend using the ISR to set a bit in a separate flag variable. Your main loop would then poll that variable; when it find that variable’s bit has been set, it can then do two things: 1) clear the bit in your flag variable (set it back to zero), and 2) print your message saying that the interrupt has occurred. Before you do that, however, I would strongly recommend that you copy the original code into your Arduino IDE and verify that everything is working properly. I tested the code on an Arduino Nano and an Arduino Uno before I posted it here, so I know it’s working correctly! Very elegant and well presented information. In a comment you made (I can’t reply to it for some reason), you said: “If you absolutely have to have serial output in your program in response to an ISR, I would recommend using the ISR to set a bit in a separate flag variable. Your main loop would then poll that variable” Doesn’t that actually cancel the hardware interrupt function concept completely??
Do you mean that the main loop should ‘monitor’ that variable? Why not monitor the pin state directly then? You’re correct that this effectively sets up the main loop to monitor another variable, and yes, it’s a slight redundancy. This sample code is a poor example of this, but interrupts are frequently used to respond to time sensitive events. Think for a moment if your embedded system were running code as a big “superloop”, with a ton of program steps executed serially inside a big loop (as opposed to nothing in this example). For some time critical applications, there’s a chance that you could miss the window in which to react to that event just due to which stage of the loop you’re in! In contrast, serial communications are actually really, really slow - the Arduino, by default, only sends 9600 ASCII characters per second.
This is way, WAY slower than a lot of stuff that the processor in the Arduino was designed to control. (For example, the internal clock is 8MHz, and the GPIO toggle rate maxes out at about 5MHz.) I guess the point I’m trying to make is that you really shouldn’t be using the serial console to signal high-speed events, as you run the risk of missing things otherwise! Guessing you’ve read the other comment threads - did you see my reply to kesarraghav?
Arduino Software Interrupt Timer
- Arduino Interrupts - Learn Arduino in simple and easy steps starting from Overview, Board Description, Installation, Program Structure, Data Types, Arrays, Passing.
- Interrupts This is a guide on implementing interrupts for your Arduino code. There is a lot of good information about interrupts out there, but this guide is part.
Printing inside of an ISR can cause serious issues with program flow, the largest one being preventing your program from returning to normal execution. Can’t thank you enough for your time. Just because my project is small and simple, I gave it a shot anyway: -An Arduino Nano samples power consumption data every 0.5sec -At a certain point it activates a digital output, to power-up an ESP8266. And keeps sampling.When the ESP8266 has connected to the MQTT broker, it issues a Hardware-Interrupt to the Arduino.Arduino serial-prints the average-Power -4 seconds later shuts-down the ESP8266. Sampling continues at all times.
Averaging gets stored at the end of every minute. It works alright for 2 days now. Probably it is too short and simple to cause issues.
Thank you for sharing the knowledge. I don’t have direct experience with the Arduino, so I’m not the best person to help you here. But it seems to me that interrupt monitoring is always an input function, regardless of whether the interrupt is sensitive to a rising edge, a falling edge, or both. The pin should not be set to OUTPUT if you want to fire an interrupt based on an external signal.
The PULLUP option simply maintains a known logic state for a pin that might otherwise be disconnected. No inversion is involved; if the pin is always driven by a logic signal or if it has an external resistor to ground or VCC, you don’t need the PULLUP option. If the pin could ever be electrically disconnected, you need either an internal pullup or an external pullup.